Basics of Flutter State Management
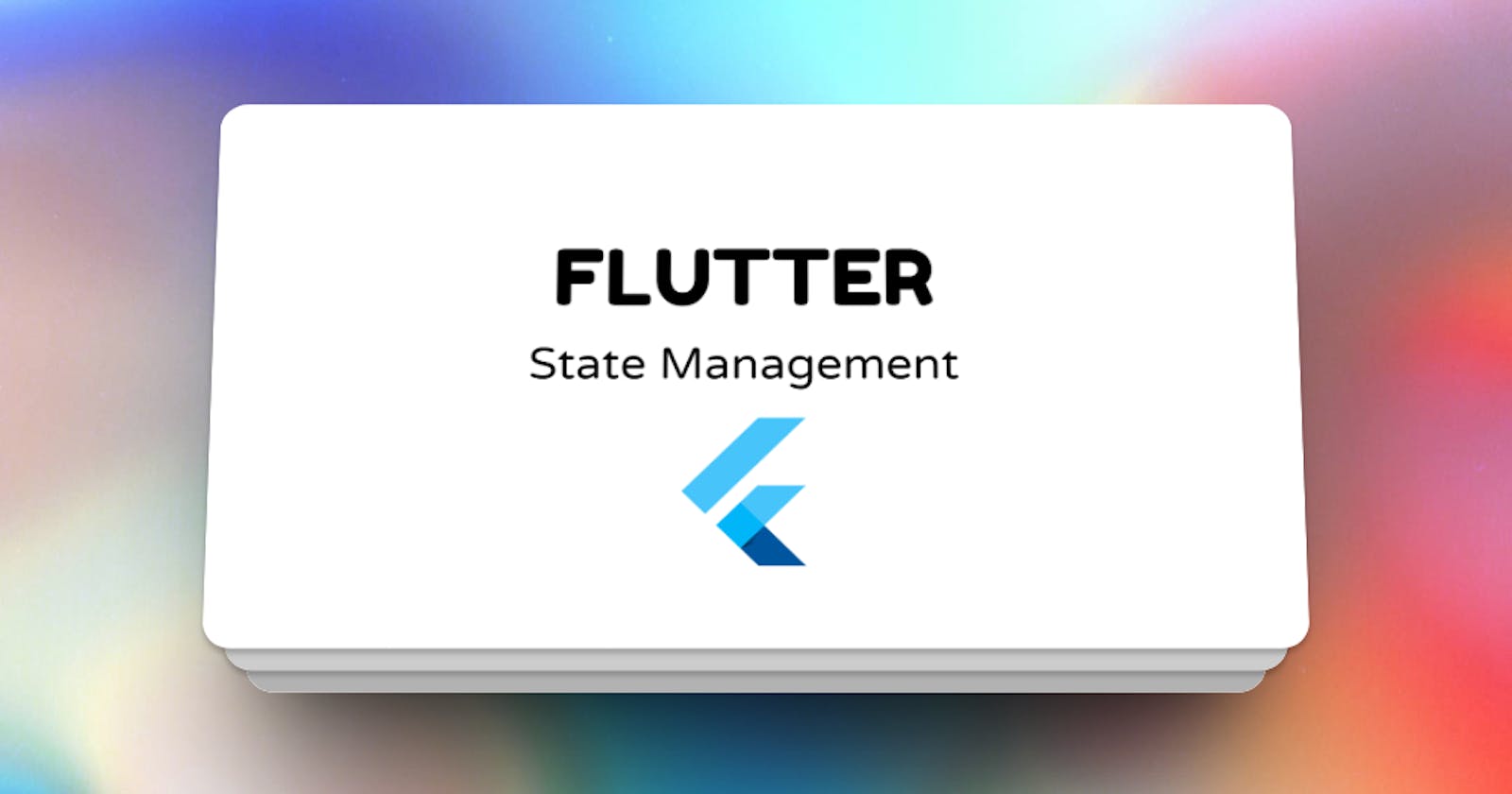
What is Flutter?
Flutter is an open-source UI framework developed by Google that enables developers to build natively compiled applications for mobile, web, and desktop from a single codebase. It offers a rich set of pre-built widgets, tools, and libraries, making it easier and faster to develop cross-platform applications.
Introduction to Flutter State Management
Flutter state management refers to the techniques and libraries used to handle and update the state of a Flutter application. It involves managing the flow of data, reacting to user interactions, and keeping the UI in sync with the underlying data.
In this article, we will explore several popular state management solutions in Flutter and discuss their features, benefits, and use cases. By understanding these options, you can choose the most suitable state management approach for your Flutter projects.
Why is State Management Important in Flutter?
State management plays a crucial role in Flutter development, especially when dealing with dynamic user interfaces and complex application logic. In Flutter, state refers to the mutable data that determine the appearance and behavior of the user interface. Managing and updating this state efficiently is essential for building responsive and interactive applications.
Common Challenges in State Management
When developing Flutter applications, developers often encounter challenges related to state management. Some of the common challenges include:
Managing Complex UI State: As applications grow in complexity, managing the state of various UI components becomes challenging.
Sharing State between Widgets: Sharing state between multiple widgets or screens requires careful design and coordination.
Handling Asynchronous Updates: Managing asynchronous updates to the state can result in race conditions and inconsistent UI behavior.
Performance Optimization: Inefficient state management can lead to unnecessary rebuilds and performance issues.
To address these challenges, Flutter provides various state management solutions, each with its own approach and advantages.
Understanding State in Flutter
In Flutter, state can be classified into two main types: local state and global state.
Local state pertains to the data that is relevant to a specific widget or subtree of the UI.
On the other hand, global state represents data that needs to be shared and accessed across multiple widgets or screens within an application.
Local State Management
Local state management focuses on managing state within a specific widget or subtree. It is suitable for scenarios where the state is not shared or accessed by other parts of the application.
InheritedWidget
InheritedWidget is a built-in Flutter class that allows for sharing data down the widget tree. It is often used as the foundation for other state management solutions. InheritedWidget allows widgets to access and update shared state efficiently, reducing unnecessary rebuilds.
ScopedModel
ScopedModel is a simple state management solution that provides a centralized model accessible to descendant widgets. It simplifies the process of sharing state across different parts of the UI hierarchy. ScopedModel follows the concept of scoped model inheritance and updates the UI whenever the model changes.
Global State Management
Global state management involves managing and sharing state across the entire application, enabling different widgets and screens to access and update the same data.
BLoC (Business Logic Component)
BLoC (Business Logic Component) is an architectural pattern that separates the UI from the business logic and state management. It relies on streams to propagate state changes and events. BLoC can be used with Flutter's built-in StreamBuilder widget to update the UI in response to state updates.
Redux
Redux is a predictable state container for Dart and Flutter applications. It follows the unidirectional data flow pattern, where actions trigger state changes through reducers. Redux provides a single store that holds the application state and ensures consistency across the entire app.
Provider
Provider is a lightweight state management solution that leverages Dart's inherited widget mechanism. It allows widgets to obtain and update state without explicitly passing it down the widget tree. Provider simplifies state management by handling the widget tree traversal and rebuilding only the necessary parts of the UI.
GetX
GetX is a powerful Flutter state management library that focuses on simplicity and performance. It offers reactive state management, dependency injection, and a wide range of utility classes and extensions. GetX provides an intuitive and concise syntax for managing and accessing state across the application.
MobX
MobX is a battle-tested state management library that embraces reactive programming. It automatically tracks observable state and updates the UI whenever the state changes. MobX minimizes boilerplate code and provides a clean and declarative approach to state management.
Riverpod
Riverpod is a provider package inspired by Provider and ScopedModel. It offers a simple and flexible API for state management and dependency injection. Riverpod focuses on improving testability and readability by avoiding global mutable state.
Hive
Hive is a lightweight and fast NoSQL database solution for Flutter. While primarily used for data persistence, Hive can also be utilized for state management. It allows for storing and accessing application state efficiently, making it suitable for small to medium-sized projects.
Firebase
Firebase is a comprehensive suite of cloud-based tools and services provided by Google. While primarily used for backend functionality, Firebase can be leveraged for real-time state synchronization and data sharing across devices. It provides Firebase Realtime Database and Cloud Firestore as options for state management in Flutter.
GraphQL
GraphQL is a query language and runtime for APIs that enables efficient data fetching and manipulation. With Flutter, GraphQL can be used for managing state by interacting with a GraphQL server. By defining GraphQL schemas and queries, Flutter apps can retrieve and update data from a centralized source.
StateNotifier
StateNotifier is a lightweight state management solution introduced in the Riverpod package. It combines the simplicity of ChangeNotifier with the predictability of StateNotifier. StateNotifier allows widgets to consume and update state in a declarative and testable manner.
Riverbloc
Riverbloc is a powerful Flutter state management library that follows the BLoC pattern. It offers improved ergonomics and testability compared to the traditional BLoC pattern. Riverbloc leverages streams and stream transformers to handle state changes and provides a convenient way to manage asynchronous events.
GetX vs. Provider
Both GetX and Provider are popular state management solutions in the Flutter ecosystem. Let's compare them based on key factors:
Feature | GetX | Provider |
Performance | Highly optimized for performance and efficient updates | Efficient, but can be slower with complex UI structures |
Simplicity | Provides a concise and intuitive syntax for state management | Requires more boilerplate code and setup |
Functionality | Offers a wide range of utility classes and extensions | Focused on providing core state management functionality |
Ecosystem | Expanding ecosystem with additional features and extensions | Well-established ecosystem with extensive community support |
Learning | Relatively easy to learn and use | Requires understanding of concepts like ChangeNotifier and BuildContext |
Documentation | Comprehensive documentation and examples | Extensive documentation and community resources available |
Redux vs. MobX
Redux and MobX are popular state management solutions that differ in their approaches. Let's compare them:
Feature | Redux | MobX |
Design | Follows unidirectional data flow and pure functions | Embraces reactive programming and observable state |
Boilerplate | Requires writing actions, reducers, and store | Provides automatic state tracking with minimal boilerplate |
Learning | Steeper learning curve due to the strict architecture | Easier to learn and adapt, especially for developers new to Flutter |
Community | Well-established community with extensive support | Strong community support and active development |
Debugging | Built-in tools for time-travel debugging and state inspection | Offers debugging tools and observability features |
Performance | Ensures predictable state updates and optimized performance | Highly performant, but complex structures can impact performance |
Flutter State Management Best Practices
When approaching state management in Flutter, consider the following best practices:
Analyze Your App's Requirements: Understand your app's state management needs before choosing a solution. Consider factors like complexity, scalability, and team familiarity.
Choose the Right Solution: Select a state management approach that aligns with your project's requirements and development style. Evaluate the pros and cons of each solution.
Separation of Concerns: Separate UI logic from business logic to ensure maintainability and testability. Follow the principle of single responsibility.
Immutable Data: Prefer immutable data structures for your application state. This helps prevent unwanted side effects and simplifies state updates.
Efficient Updates: Optimize state updates by using granular updates, such as using
setState
for local state andValueNotifier
for small and localized changes.Testing: Write unit tests for your state management code to ensure its correctness and maintainability. Mock dependencies and cover edge cases.
Documentation: Document your state management approach and the reasoning behind it. This helps new developers onboard smoothly and promotes code comprehension.
Conclusion
State management is a critical aspect of Flutter development, enabling efficient handling of application state and ensuring responsive user experiences. In this article, we explored various state management solutions in Flutter, including local and global state management approaches. We compared popular libraries like BLoC, Redux, Provider, GetX, MobX, Riverpod, Hive, Firebase, GraphQL, StateNotifier, and Riverbloc.
By understanding the features, benefits, and trade-offs of each solution, you can make an informed decision about the best state management approach for your Flutter projects. Remember to follow best practices, analyze your app's requirements, and choose a solution that aligns with your project's needs.
Thanks for reading 🫡
If you want to read more such articles, Visit DevelopnSolve.
Frequently Asked Questions
Q1: What is state management in Flutter?
State management in Flutter refers to the techniques and libraries used to handle and update the state of a Flutter application. It involves managing the flow of data, reacting to user interactions, and keeping the UI in sync with the underlying data.
Q2: Why do we need state management in Flutter?
State management is crucial in Flutter to handle dynamic user interfaces and complex application logic. It allows developers to manage and update the state efficiently, ensuring responsive and interactive user experiences.
Q3: What are the common challenges in state management?
Common challenges in state management include managing complex UI state, sharing state between widgets, handling asynchronous updates, and optimizing performance to prevent unnecessary rebuilds.
Q4: What are some popular state management solutions in Flutter?
Some popular state management solutions in Flutter include BLoC, Redux, Provider, GetX, MobX, Riverpod, Hive, Firebase, GraphQL, and StateNotifier.
Q5: Which state management solution is best for my Flutter project?
The best state management solution depends on various factors such as project complexity, team familiarity, performance requirements, and personal preference. Evaluate the features, advantages, and trade-offs of each solution to choose the one that suits your project best.
Q6: What are the best practices for Flutter state management?
Some best practices for Flutter state management include analyzing your app's requirements, choosing the right solution, separating concerns, using immutable data, optimizing state updates, writing tests, and documenting your state management approach.