Best Practices for Code Documentation
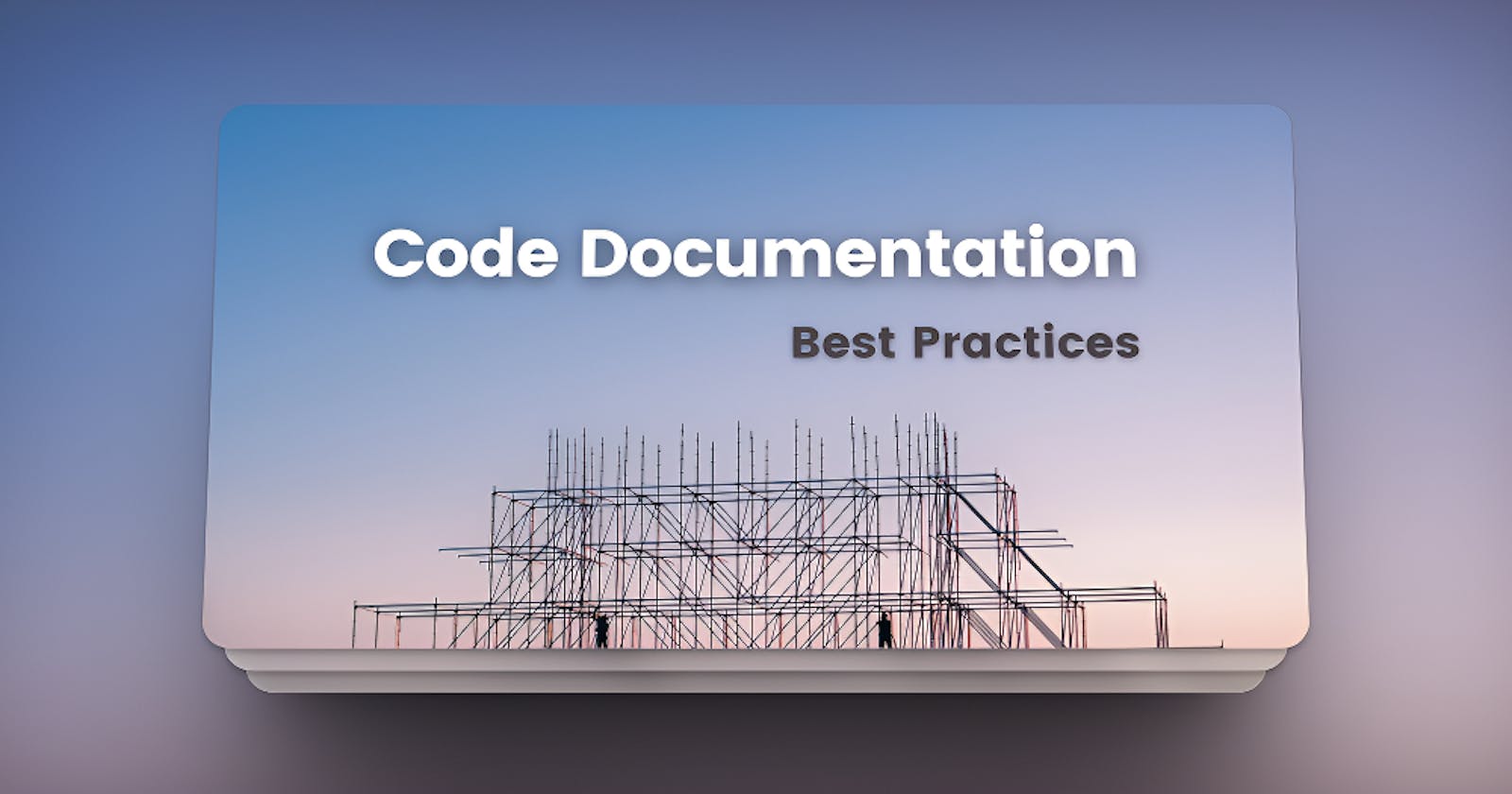
Introduction
Code documentation is an essential aspect of software development that often gets overlooked. Proper documentation helps developers understand the purpose and functionality of the code, facilitates collaboration among team members, and ensures the maintainability of the software over time.
In this article, we will see and try to understand the best practices for code documentation that can greatly improve the quality and readability of your codebase.
Why is Code Documentation Important?
Before delving into the best practices, let's first understand why code documentation is important. Here are a few key reasons:
Enhances Code Understandability: Well-documented code makes it easier for developers to understand its purpose and functionality. It serves as a guide, helping them navigate through complex codebases.
Facilitates Collaboration: Documentation acts as a common language among team members, allowing them to work together seamlessly. It provides context, making it easier for others to contribute to the codebase.
Improves Maintainability: As software evolves, it undergoes changes and updates. Proper documentation enables developers to quickly identify and modify the relevant sections, reducing the chances of introducing bugs or breaking existing functionality.
Simplifies Onboarding: When new developers join a project, they often struggle to understand the codebase. Documentation eases the learning curve and accelerates their productivity.
Best Practices for Code Documentation
Now that we understand the importance of code documentation, let's dive into some best practices that can help you create effective documentation.
1. Document the Purpose of the Code
Every piece of code should have a clear purpose. Documenting the purpose of the code helps other developers understand its intent. Use comments to provide a brief explanation of what the code does and why it exists.
Example:
# This function calculates the average of a list of numbers.
def calculate_average(numbers):
...
2. Use Descriptive Variable and Function Names
Choosing meaningful and descriptive names for variables, functions, and classes eliminates the need for excessive comments. Well-named entities make the code self-explanatory and reduce the cognitive load for developers.
3. Provide Function and Method Documentation
For complex functions or methods, provide a detailed explanation of what they do, the expected inputs, and the return values. Use docstrings to document the function signatures, arguments, and the purpose of the function.
Example:
def calculate_average(numbers):
"""
Calculates the average of a list of numbers.
Args:
numbers (List[float]): A list of numbers.
Returns:
float: The average of the numbers.
"""
...
4. Document Edge Cases and Assumptions
Code often has to handle edge cases or relies on certain assumptions. Clearly document these cases and assumptions to guide future developers and prevent potential issues.
5. Use Markdown for Formatting
Markdown is a simple and widely supported markup language that allows you to format your documentation effectively. You can use Markdown to create headings, lists, tables, and add emphasis to text.
Example:
#### Example Heading
- Item 1
- Item 2
| Column 1 | Column 2 |
| -------- | -------- |
| Data 1 | Data 2 |
6. Update Documentation Alongside Code Changes
Documentation should evolve with the codebase. Whenever you make changes to the code, ensure that you update the corresponding documentation. Outdated documentation can lead to confusion and errors.
7. Use Diagrams and Flowcharts
Visual representations, such as diagrams and flowcharts, can greatly enhance the understanding of complex code. Use tools like draw.io or Lucid chart to create visualizations that illustrate the code's flow or architecture.
8. Include Usage Examples
Provide usage examples and code snippets to demonstrate how to use your code. This helps other developers quickly grasp the functionality and usage patterns.
9. Use Version Control to Track Documentation Changes
Just like code, documentation should be versioned and stored in a version control system. This allows you to track changes, revert to previous versions, and collaborate effectively with other team members.
10. Leverage Inline Comments Sparingly
While comments are valuable, excessive commenting can clutter the code and reduce readability. Use inline comments sparingly and only when necessary to explain complex logic or provide additional context.
11. Document Dependencies and Installation Steps
If your code depends on external libraries or has specific installation steps, clearly document these requirements. This helps other developers set up the project and ensures a smooth development experience.
12. Keep Documentation Concise and Readable
Avoid writing lengthy paragraphs and aim for concise documentation that conveys information effectively. Use bullet points, headings, and subheadings to structure the documentation for easy scanning and reading.
13. Document APIs and Interfaces
When developing libraries or frameworks, document the public APIs and interfaces thoroughly. This allows users of your code to understand how to interact with it and leverage its capabilities.
14. Consider User Feedback and Questions
User feedback and questions can provide valuable insights into areas where your code documentation may be lacking. Pay attention to user queries and incorporate improvements based on their suggestions.
15. Automate Documentation Generation
Explore tools and frameworks that automate the generation of documentation from your codebase. These tools can extract information from code comments and generate documentation in various formats, such as HTML or PDF.
16. Follow Established Documentation Standards
If your codebase follows a particular programming language or framework, adhere to the established documentation standards for that ecosystem. This ensures consistency across projects and familiarity for developers.
17. Document Error Handling and Exceptions
In addition to documenting the expected behavior of your code, also document how errors and exceptions are handled. Describe the types of errors that can occur and provide guidance on handling or troubleshooting them.
18. Include References and External Links
When applicable, include references to external resources and relevant documentation that can provide additional context or in-depth explanations. These references help readers explore the topic further and gain a deeper understanding.
19. Collaborate on Documentation
Consider documentation as a collaborative effort and involve multiple team members in its creation. Different perspectives can lead to better documentation quality and a broader understanding of the codebase.
20. Regularly Review and Update Documentation
Documentation is not a one-time task. Schedule regular reviews of the documentation to ensure it remains up-to-date and relevant. Make it a team responsibility to keep the documentation accurate and comprehensive.
Conclusion
Effective code documentation is crucial for maintaining software quality and enabling collaboration among developers. By following the best practices outlined in this article, you can create documentation that enhances code understandability, simplifies collaboration, and improves software maintainability.
Remember to keep your documentation concise, up-to-date, and accessible to other team members. Embrace the collaborative nature of documentation and continuously strive to improve its quality. Happy documenting!
Thanks for reading 🫡, See you in the article.
FAQs
Q: How much documentation is too much?
A: Documentation should strike a balance between being comprehensive and concise. Avoid unnecessary verbosity and focus on providing essential information that aids in understanding and using the code.
Q: Should I document every line of code?
A: Documenting every line of code is usually excessive. Instead, focus on documenting complex logic, important assumptions, and any potential pitfalls or edge cases.
Q: Is it better to have inline comments or separate documentation files?
A: Inline comments are helpful for explaining specific lines or sections of code, while separate documentation files provide a higher-level overview of the codebase. Use both approaches judiciously based on the context.
Q: How frequently should I update the documentation?
A: Ideally, documentation should be updated alongside code changes. However, at a minimum, schedule regular reviews to ensure the documentation remains accurate and up-to-date.
Q: Can I rely solely on automated documentation generation tools?
A: Automated documentation generation tools can be a useful starting point, but it's important to review and enhance the generated documentation manually. Human intervention ensures clarity, correctness, and completeness.
Q: What if I inherit a codebase with no documentation?
A: Inheriting a codebase without documentation can be challenging. Start by documenting the most critical sections first and gradually expand the documentation as you gain a deeper understanding of the code.