Java Regex 101 - Mastering the Basics
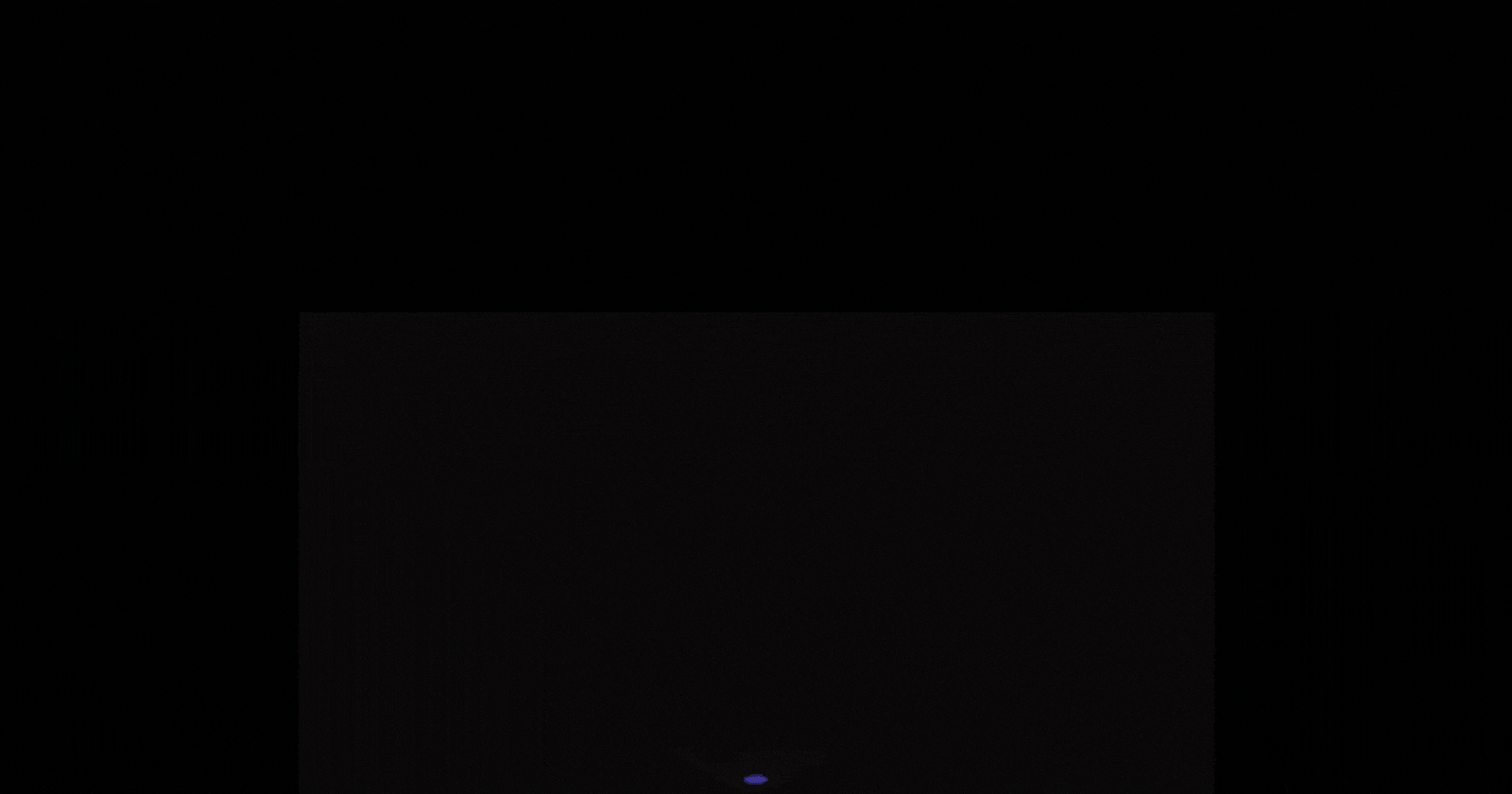
Introduction
Regular expressions, also known as RegEx, are an essential tool for pattern matching and data validation in programming languages. They offer a versatile and compact syntax for detecting and modifying text patterns. Java programmers can leverage the java.util.regex
package, which provides various classes and methods for working with regular expressions.
In this post, we will explore the fundamentals of RegEx syntax, advanced functionalities, real-world examples, as well as tools and libraries that you can use to simplify the process of working with regular expressions. We will also share some helpful tips and tricks, and best practices for crafting robust and efficient regular expressions. Whether you're new to RegEx or a seasoned expert, this article will equip you with the knowledge and skills you need to take your RegEx proficiency to the next level.
So without wasting any time, Let's get into this.
Basic Syntax
The building blocks of regular expressions are character classes and sets, anchors and boundaries, quantifiers and repetition, as well as alternation (OR operator). These elements form the foundation of RegEx syntax, allowing you to identify and manipulate text patterns with ease.
Understanding the basic syntax of regular expressions is crucial for effectively leveraging their power and flexibility. In the following sections, we will delve deeper into each of these elements and explore how they can be combined to create powerful and effective RegEx patterns.
Character classes and sets
Character classes and sets are essential components of regular expressions that enable you to match specific characters or groups of characters. By using character classes, you can define a set of characters that a pattern can match.
For example, you can use the character class [abc]
to match any single character that is either a, b, or c
. This is useful when you need to match specific characters within a larger pattern.
In addition to defining your own character sets, regular expressions provide shorthand character classes to match commonly used character sets. For instance, the \d
character class matches any digit character (0-9), and the \s
character class matches any whitespace character, including spaces, tabs, and newlines.
By utilizing character classes and sets, you can create more precise and efficient regular expressions that accurately match your desired patterns.
Here are some examples of character classes and sets :
[abc] - matches any single character that is either a, b, or c
[^abc] - matches any single character that is not a, b, or c
[a-z] - matches any single lowercase letter from a to z
[A-Z] - matches any single uppercase letter from A to Z
[a-zA-Z] - matches any single letter, either lowercase or uppercase
[0-9] - matches any single digit character from 0 to 9
[^0-9] - matches any single character that is not a digit
[\s] - matches any single whitespace character, including spaces, tabs, and newlines
[\S] - matches any single non-whitespace character
[\d] - matches any single digit character (equivalent to [0-9])
[\D] - matches any single non-digit character
Anchors and boundaries
Anchors and boundaries are critical elements of regular expressions that help you match patterns positioned at the beginning or end of a line, word, or string. With anchors, you can ensure that a pattern only matches at a specific position within a string.
For instance, the ^
anchor matches the beginning of a line, and the $
anchor matches the end of a line. This allows you to match patterns that occur at the start or end of a line, such as the first or last word in a sentence.
In addition to anchors, regular expressions provide boundaries that match the boundaries of words or non-word characters. The \b
boundary matches the word boundary, and the \B
boundary matches the non-word boundary. This can be useful when you need to match patterns that are surrounded by specific characters, such as matching a whole word but not a partial match.
Here are some examples of Anchors and Boundaries :
^ - Matches the beginning of a line or string.
$ - Matches the end of a line or string.
\b - Matches a position between a word character (as defined by \w) and a non-word character (as defined by \W).
\B - Matches a position that is not a word boundary.
\A - Matches the beginning of a string (but not the beginning of a line).
\G - Matches the end of the previous match.
\Z - Matches the end of a string or the position just before a newline at the end of a string.
\z - Matches the absolute end of a string.
Quantifiers and repetition
Quantifiers and repetition are crucial elements of regular expressions that enable you to match patterns that occur zero or more times, one or more times, or a specific number of times. By using quantifiers and repetition, you can create more flexible and powerful patterns.
For instance, the *
quantifier matches zero or more occurrences of the previous pattern, while the +
quantifier matches one or more occurrences of the previous pattern. This can be useful when you need to match patterns that occur multiple times in a row, such as matching multiple whitespace characters.
You can also use curly braces to specify a specific number of occurrences. For example, the {3}
quantifier matches exactly three occurrences of the previous pattern, while the {3,5}
quantifier matches between three and five occurrences of the previous pattern. This is particularly useful when you need to match a specific number of characters, such as matching a phone number with a fixed number of digits.
Alternation (OR operator)
Alternation, also known as the OR operator, is a powerful tool in regular expressions that enables you to match patterns that are either one pattern or another pattern. With alternation, you can define multiple options for a pattern and match any of those options.
For example, the (red|blue|green)
pattern matches any string that contains the words red, blue, or green. This can be useful when you need to match patterns that can have different variations or options, such as matching different spellings of a word or different formats of a phone number.
Using alternation, you can create more versatile and comprehensive regular expressions that can match a wider range of patterns.
Advanced Syntax
The advanced syntax of regular expressions includes lookaround assertions, backreferences and subexpressions, and greedy vs. lazy matching.
Lookaround assertions
Lookaround assertions are a powerful feature in regular expressions that enable you to match patterns based on what comes before or after them, without actually including that text in the match. Lookaround assertions are useful when you need to match patterns that are only valid under certain conditions or when you need to exclude certain patterns from a match.
There are two types of lookaround assertions: positive lookaround and negative lookaround.
Positive lookaround uses the (?=pattern)
syntax to match patterns that are followed by the specified pattern. For example, the pattern (?=jane)
will match any string that is followed by the word "jane". In other words, it will match a pattern only if it is immediately followed by another pattern.
Negative lookaround, on the other hand, uses the (?!pattern)
syntax to match patterns that are not followed by the specified pattern. For example, the pattern (?!john)
will match any string that is not followed by the word "john". Negative lookaround is useful when you want to match a pattern only if it is not followed by a certain pattern.
Both positive and negative lookaround assertions can be used in combination with other regular expression syntax to create more complex and specific pattern matches.
Backreferences and subexpressions
Backreferences and subexpressions are two advanced features in regular expressions that allow you to reuse parts of a pattern in the same expression or in the replacement string of a search and replace operation.
Backreferences are created by using the (\d)
syntax to capture a specific group of digits and then reference that group later in the expression using the backslash followed by the group number. For example, the pattern (\d)\1
matches any two consecutive digits that are the same. Here, the parentheses capture the first digit, and the \1
backreference references that same digit in the second position. This means that the pattern will match any string that contains two identical consecutive digits.
Subexpressions, on the other hand, use the ()
syntax to group patterns together and apply operations to the group as a whole. For example, the pattern (red|blue)
car matches any string that contains either the phrase "red car" or "blue car". Here, the parentheses group the two color options together, and the vertical bar separates the two options. This allows you to match multiple patterns at once and create more complex pattern matching expressions.
Both backreferences and subexpressions are powerful tools that can greatly increase the flexibility and effectiveness of regular expressions. They can be used to create more sophisticated pattern matches and streamline the search and replace process.
Greedy & lazy matching
When it comes to matching patterns, regular expressions offer two different matching styles: greedy and lazy. The default behavior is greedy matching, where the engine matches the longest possible string that matches the pattern. This means that if you use the pattern .*
to match any string, the engine will try to match as many characters as possible, even if that means matching past the desired end point.
On the other hand, lazy matching matches the shortest possible string that matches the pattern. This style of matching can be useful in situations where you need to match a specific pattern within a larger string, and you want to avoid matching past the desired end point. You can specify lazy matching by using the ?
operator, like this: .*?
. This pattern will match the shortest possible string that contains any number of characters.
It's important to keep in mind that lazy matching can sometimes lead to unexpected results, especially when working with complex patterns. In general, it's best to use greedy matching by default, and only switch to lazy matching when you specifically need to match the shortest possible string.
Practical Examples
Now let's look at some practical examples of regular expressions in Java.
Validation for email addresses, phone numbers, and passwords
Regular expressions can be used to validate user input for email addresses, phone numbers, and passwords. For example:
// Email address validation
String emailRegex = "^[A-Za-z0-9+_.-]+@[A-Za-z0-9.-]+$";
String email = "example@email.com";
if (email.matches(emailRegex)) {
System.out.println("Valid email address");
} else {
System.out.println("Invalid email address");
}
// Phone number validation
String phoneRegex = "^\\d{3}-\\d{3}-\\d{4}$";
String phone = "123-456-7890";
if (phone.matches(phoneRegex)) {
System.out.println("Valid phone number");
} else {
System.out.println("Invalid phone number");
}
// Password validation
String passwordRegex = "^(?=.*[0-9])(?=.*[a-z])(?=.*[A-Z])(?=.*[@#$%^&+=])(?=\\S+$).{8,}$";
String password = "Abcd@1234";
if (password.matches(passwordRegex)) {
System.out.println("Valid password");
} else {
System.out.println("Invalid password");
}
Filtering and matching text patterns in programming languages
Regular expressions can be used to filter and match text patterns in programming languages. For example, you can use regular expressions to search for specific strings or patterns in a file or string:
// Search for a pattern in a string
String str = "The quick brown fox jumps over the lazy dog";
String pattern = "brown";
if (str.matches(".*" + pattern + ".*")) {
System.out.println("Pattern found");
} else {
System.out.println("Pattern not found");
}
// Replace a pattern in a string
String newStr = str.replaceAll(pattern, "red");
System.out.println(newStr);
Search and replace using regular expressions
Regular expressions can also be used for search and replace operations, where you can replace a pattern with another pattern. For example:
// Replace all vowels with an asterisk
String str = "Hello, World!";
String newStr = str.replaceAll("[AEIOUaeiou]", "*");
System.out.println(newStr);
// Replace all whitespace characters with a dash
String str2 = "This is a sentence.";
String newStr2 = str2.replaceAll("\\s", "-");
System.out.println(newStr2);
Tools and Libraries
There are many tools and libraries available for working with regular expressions in Java.
Popular tools, software, and plugins that support RegEx
Some popular tools and software that support regular expressions include:
Notepad++
Visual Studio Code
IntelliJ IDEA
Eclipse
Overview of RegEx libraries in different programming languages
Different programming languages have their own regular expression libraries. Some of the popular libraries include:
java.util.regex
(Java)re
(Python)regex
(Rust)std::regex
(C++)re2
(C++)
Exploring RegEx live debuggers and testers
There are many online tools available for testing and debugging regular expressions. Some popular tools include:
RegExr
Regex101
RegEx Pal
These tools allow you to enter a regular expression and test it against different input strings.
Tips and Tricks
Here are some tips and tricks for mastering regular expressions:
Dos and Don'ts of RegEx
Do use character classes and sets to match specific characters or ranges of characters.
Do use anchors and boundaries to match at the beginning or end of a line or word.
Do use quantifiers and repetition to match patterns of characters.
Do use alternation to match one of several possible patterns.
Don't use regular expressions for tasks that can be easily accomplished with simpler string manipulation functions.
Don't overuse greedy matching, as it can lead to unexpected results.
Don't use regular expressions for tasks that require complex parsing or matching beyond what is supported by regular expressions.
Common mistakes and how to avoid them
Forgetting to escape special characters: Special characters like
^
,$
,*
,+
,?
,.
,|
,(
,)
,[
,]
,{
, and}
have special meanings in regular expressions, so you need to escape them with a backslash (\
) to match them literally.Overcomplicating patterns: Regular expressions can quickly become complex, so it's important to keep patterns simple and easy to read and maintain.
Not testing patterns thoroughly: Always test your regular expressions with different input strings to make sure they match the intended patterns and don't produce unexpected results.
Best practices when writing regular expressions
Use comments to explain complex patterns: Regular expressions can quickly become hard to read, so it's a good idea to use comments to explain complex patterns and make them easier to understand and maintain.
Use tools and libraries to simplify pattern matching: Many programming languages provide built-in functions and libraries for pattern matching, which can simplify regular expression patterns and make them easier to read and maintain.
Optimize patterns for performance: Regular expressions can be computationally expensive, so it's important to optimize patterns for performance by avoiding redundant or unnecessary operations.
Conclusion
Regular expressions are a vital tool in a programmer's toolbox for working with text patterns in languages like Java. By understanding both the basic and advanced syntax of regular expressions, you can easily validate user input, filter and match text patterns, and even perform search and replace operations. With regular expressions, you can write code that is both efficient and robust.
To become a master of regular expressions, it's crucial to learn and implement best practices while working with them. This includes understanding when to use lazy vs. greedy matching, properly using lookaround assertions and subexpressions, and ensuring that your regular expressions are optimized for performance.
By following these best practices, you can make your code more maintainable and efficient. With regular expressions, you can manipulate and extract the information you need from a given input text, making your programs more powerful and versatile.
Thanks for Reading.
See you in the next blog, Until then keep developing and solving.
Comments (12)
Really helpful Pratik Mali
Great article Pratik Mali
detailed blogpost ever
well explained
Well written Pratik Mali
Thanks for sharing Pratik Mali
ell-organized introduction to regular expressions in Java Pratik Mali
examples provided are helpful and practicalPratik Mali
explanations of more advanced topics are clear and concise Pratik Mali
way you explained the syntax and structure of regex was on point Pratik Mali
well explained